Examples
Configure Basic introduction
Via the `products/configure` endpoint you can configure Probo products "on the fly" via a step-by-step approach, without the need to build a custom validation mechanism as you would need to make when using the `/products/product/:code` endpoint. It is notable to mention, that you still need to bring your front end and handle the rendering.
Step 1; Initial options.
To get started with the configuration you need a Probo product code. You can retrieve this on the product page on the Probo platform or via the GET /products
endpoint.
You use the product code to get the first step of the configuration process. In our example we will use Dibond. We will use this in our POST call to the /products/configure
endpoint.
First call to get the options
{
"language" : "en",
"products": [
{
"code": "dibond"
}
]
}
Response
{
"status": "ok",
"code": 200,
"message": "Options calculated",
"products": [
{
"id": 1,
"code": "dibond",
"can_order": false,
"amount": 0,
"width": 0,
"height": 0,
"length": 0,
"available_options": [
{
"name": "Size",
"label": null,
"code": "size",
"can_order": null,
"amount": null,
"width": null,
"height": null,
"length": null,
"available": true,
"unit_code": null,
"price": null,
"children": [
{
"type_code": "width",
"name": "Width",
"label": null,
"description": null,
"value": null,
"code": "width",
"default_value": null,
"min_value": "7.00",
"max_value": "99999.00",
"step_size": null,
"scale": 1,
"reversible": true,
"last_option": false,
"available": true,
"unit_code": "cm",
"price": null,
"images": []
},
{
"type_code": "height",
"name": "Height",
"label": null,
"description": null,
"value": null,
"code": "height",
"default_value": null,
"min_value": "5.00",
"max_value": "99999.00",
"step_size": null,
"scale": 1,
"reversible": true,
"last_option": false,
"available": true,
"unit_code": "cm",
"price": null,
"images": []
}
]
},
{
"name": "Amount",
"label": null,
"code": "amount",
"can_order": null,
"amount": null,
"width": null,
"height": null,
"length": null,
"available": true,
"unit_code": null,
"price": null,
"children": [
{
"type_code": "amount",
"name": "Amount",
"label": null,
"description": null,
"value": null,
"code": "amount",
"default_value": null,
"min_value": null,
"max_value": null,
"step_size": null,
"scale": null,
"reversible": null,
"last_option": false,
"available": true,
"unit_code": "pc",
"price": null,
"images": []
}
]
}
],
"selected_options": []
}
]
}
Breakdown.
After the first call, we will get an object a with a child property available_options
. This is the first step of the product configuration. We can use this to ask for user input and prepare the second step. How you render the front end is out of scope
The selected options need to be passed along to get to the next step. For this, we use the code from each child in the first step ( available_options[].childeren[].code
)
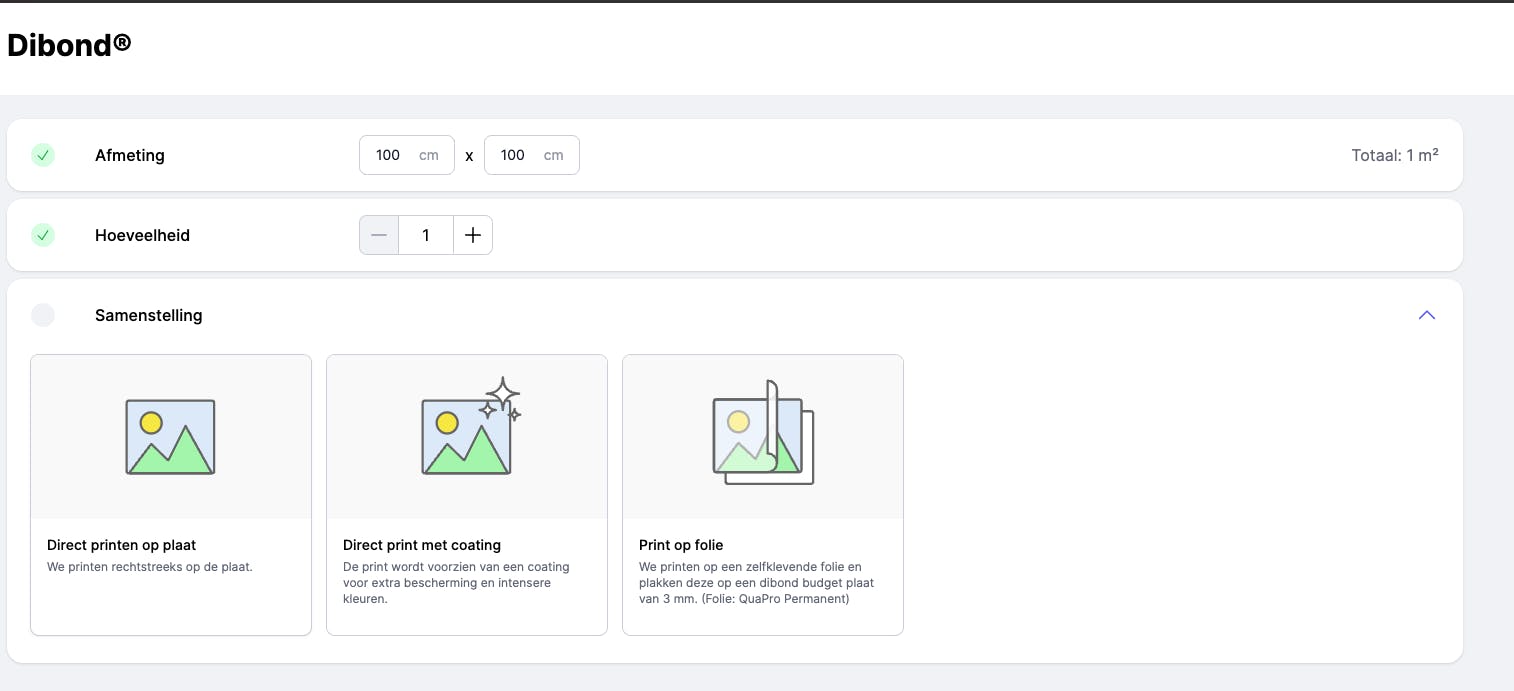
Second step to get the next step
{
"language" : "en",
"products": [
{
"code": "dibond",
"options": [
{
"code": "width",
"value": "100"
},
{
"code": "height",
"value": "100"
},{
"code" : "amount",
"value":"2"
}
]
}
]
}
Response Second step to get the next step
{
"status": "ok",
"code": 200,
"message": "Options calculated",
"products": [
{
"id": 1,
"code": "dibond",
"can_order": false,
"amount": 2,
"width": 100,
"height": 100,
"length": 0,
"available_options": [
{
"name": "Composition",
"label": null,
"code": "print-material",
"can_order": null,
"amount": null,
"width": null,
"height": null,
"length": null,
"available": true,
"unit_code": null,
"price": null,
"children": [
{
"type_code": "radio",
"name": "Direct-to-plate print",
"label": null,
"description": "We print directly on the plate.",
"value": null,
"code": "direct-to-plate",
"default_value": null,
"min_value": null,
"max_value": null,
"step_size": null,
"scale": null,
"reversible": null,
"last_option": false,
"available": true,
"unit_code": null,
"price": null,
"images": [
{
"language": "all",
"url": "https://cdn.print-uploader.com/201808/1529/4a7e94c2e6723434.svg"
}
]
},
{
"type_code": "radio",
"name": "Direct print with coating",
"label": null,
"description": "The print is provided with a coating for extra protection and more intense colors.",
"value": null,
"code": "seal-coating",
"default_value": null,
"min_value": null,
"max_value": null,
"step_size": null,
"scale": null,
"reversible": null,
"last_option": false,
"available": true,
"unit_code": null,
"price": null,
"images": [
{
"language": "nl",
"url": "https://cdn.print-uploader.com/202205/6025/21a4672928d92463.svg"
},
{
"language": "de",
"url": "https://cdn.print-uploader.com/202205/6026/45340fbcf022996a.svg"
}
]
},
{
"type_code": "radio",
"name": "Print on foil",
"label": null,
"description": "We print on a self-adhesive film and apply it to a 3mm dibond budget panel.",
"value": null,
"code": "print-on-film",
"default_value": null,
"min_value": null,
"max_value": null,
"step_size": null,
"scale": null,
"reversible": null,
"last_option": false,
"available": true,
"unit_code": null,
"price": null,
"images": [
{
"language": "all",
"url": "https://cdn.print-uploader.com/201808/1530/a159b83aaee437c5.svg"
}
]
}
]
}
],
"selected_options": [
{
"type_code": "width",
"name": "Width",
"label": null,
"description": null,
"value": "100",
"code": "width",
"default_value": null,
"min_value": "7.00",
"max_value": "99999.00",
"step_size": null,
"scale": 1,
"reversible": true,
"parent_code": "size",
"parent_name": "Size",
"unit_code": "cm",
"images": []
},
{
"type_code": "height",
"name": "Height",
"label": null,
"description": null,
"value": "100",
"code": "height",
"default_value": null,
"min_value": "5.00",
"max_value": "99999.00",
"step_size": null,
"scale": 1,
"reversible": true,
"parent_code": "size",
"parent_name": "Size",
"unit_code": "cm",
"images": []
},
{
"type_code": "amount",
"name": "Amount",
"label": null,
"description": null,
"value": "2",
"code": "amount",
"default_value": null,
"min_value": null,
"max_value": null,
"step_size": null,
"scale": null,
"reversible": null,
"parent_code": "amount",
"parent_name": "Amount",
"unit_code": "pc",
"images": []
}
]
}
]
}
After the call, we get the next steps but also the selected options. If we repeat the next step 2. We can push our next choice. Because the type_code is "radio" we only have to send the code.
We can repeat these steps until we get can_order : true
on the product object. The property can_order
indicates if the configuration is complete so we order the product.
Things to consider
- You should store the Probo product code in your local db identity. For example Woocommerce Product or Magento Product
- Due to CORS protection you cannot call the API via the front-end and you should create a proxy script to relay the calls to the Probo API.